
Third-Party Plugins
Custom Installations
Embedding in Documents
Installation
FastComments is designed to be installed on any kind of page - static or dynamic, light themed or dark, public or internal pages. It should be easy to install and adapt to any kind of site or web based application.
Wordpress 
You can find our WordPress plugin here.
A complete installation guide and docs around the plugin are here.
This plugin supports live commenting, SSO, and no-code installation. Simply follow the installation guide in the admin page after installing the plugin. It will guide you through connecting your WordPress installation to your account.
Any comments left with FastComments through our WordPress plugin can be automatically synced back to your WordPress install so that you retain control over your data. This can be turned off to limit the size of your WordPress database.
VanillaJS / HTML Snippet 
The VanillaJS version of the widget is very easy to install, not requiring any build systems or server side code.
Simply add the following code snippet to any page:


You can use the same code snippet on many pages; it will automatically create a separate thread per page.
Many applications have an "HTML Embed Code" option. Select that and paste the code snippet above in.
You also don't need an account to try it! You might see "tenantId: demo" in the above snippet if you're not logged in. This way it will use the demo account.
You can find documentation on configuring the widget here.
All versions of the FastComments widget are wrappers around the core VanillaJS library. This allows us to add features and fix issues in one place - and the changes automatically propagate to the other variants of the commenting widget.
Angular 
You can find our Angular library on NPM here.
The FastComments Angular commenting widget supports all of the same features of the VanillaJS one - live commenting, sso, and so on.
You will need fastcomments-typescript, which is a peer dependency. Please ensure this is included in your TypeScript compilation. In the future, this peer dependency will be moved to @types/fastcomments which will simplify this installation.

The peer dependency should be added in your tsconfig.json file, for example:

Then, add the FastCommentsModule
to your application:

Usage
To get started, we pass a config object for the demo tenant:

Since the configuration can get quite complicated, we can pass in an object reference:


The widget uses change detection, so changing any properties of the configuration object will cause it to be reloaded.
You can find the configuration the Angular component supports here.
React 
You can find our React library on NPM here.
The FastComments React commenting widget supports all of the same features of the VanillaJS one - live commenting, sso, and so on.



If you're in the EU, you'll want to set the region
parameter like so:

You can find the configuration the React component supports here.
React Native 
You can find our React Native library on NPM here.
The FastComments React Native commenting widget supports all of the same features of the VanillaJS one - live commenting, sso, and so on.


The configuration is specified slightly differently compared to the fastcomments-react
library:

If you're in the EU, you'll want to set the region
parameter:

You can find the configuration the React Native component supports here.
Vue 
You can find our Vue library on NPM here.
Additionally, a vue-next library is on NPM here
The source code can be found on GitHub.
The FastComments Vue commenting widget supports all of the same features of the VanillaJS one - live commenting, sso, and so on.
The below instructions are for Vue 3 since it has been out for some time, however FastComments also supports Vue 2 via the fastcomments-vue
library.



If you're in the EU, you'll want to set the region
to EU
:

The fastcomments-vue
and fastcomments-vue-next
libraries support the same configuration as the VanillaJS commenting widget.
You can find the configuration the Vue component supports here.
Multiple Instances on The Same Page 
Each instance of the comment widget is isolated. Because of this, FastComments inherently supports more than one instance per page, or multiple instances pointing to the same chat thread.
In the case of the VanillaJS library for example, you simply have to tie the comment widget to different DOM nodes. If you want to simply update the current thread on the page, see Switching Comment Threads Without Reloading The Page;
Syncing Authentication State Across Multiple Instances
Let's go over the example of a custom single-page-application that is a list of frequently asked questions with their own comment thread.
In this case, we have multiple instances of FastComments in the DOM at once.
This is fine, but it poses some challenges for user experience.
Consider this flow:
- The user visits the page with a list of questions, each with their own comment widget.
- The user enters their username and email and leaves a question on one of the threads.
- They see another FAQ item they have a question about.
- They go to comment again. Do they have to enter their email and username again?
In this case, FastComments handles syncing the authentication state across widget instances for you. In step four, the user will already be temporarily authenticated since they entered their username and email on the same page.
Common Use Cases 
Showing Live Comments Right Away
The comment widget is live by default, however live comments appear under a "Show N New Comments" button to prevent the page content from moving around.
In some cases, it's still desirable to show the new comments right away, without having to click a button.
In this case, you want to enable the showLiveRightAway
flag, which you can find documentation for here.
Allowing Anonymous Commenting (Not Requiring Email)
By default, FastComments requires the user to leave an email when they comment.
This can be disabled, instructions are here.
Custom Styling
Many of our customers apply their own styling to the comment widget. You can find the documentation here.
Showing The Same Comments on Multiple Domains
Showing the same comments on multiple sites is something FastComments supports out of the box. See our documentation on this subject.
Changing The Current Page
FastComments supports SPAs and complex applications. Changing the current page is easy, and covered here.
Debugging Common Issues 
Here are some symptoms we see encountered frequently, and common solutions.
"This is a demo" Message
This is shown when you've copied the widget code from our home page, which uses our demo tenant. To use your tenant, copy the widget code from here.
"FastComments cannot load on this domain" Error
FastComments needs to know which domains are owned by you to authenticate requests associated with your account. Check out our documentation to see how to resolve this error (simply add the exact subdomain + domain to your account).
Note that this should only occur after the trial period is over. During the trial period, any requests from new domains will automatically be added to your account.
Migrated Comments Not Showing for Custom Installations
Usually this happens when the imported comments are tied to a Page ID
, and you are passing a URL
(or no value, in which case it defaults to the page URL).
You can debug this by exporting your comments and viewing the URL ID
column (currently Column B
).
Ensure the values you see in the URL ID
column are the same values you are passing to the widget
configuration as the urlId
parameter.
For further explanation, try reading our How Comments are Tied to Pages and Articles documentation.
If all else fails, reach out to us.
Comment Widget Not Showing
If the comment widget isn't showing, check the Chrome developer console for errors.
For most misconfiguration, the comment widget will at least show an error on the page if it is able to load. Seeing nothing is usually an indication of a scripting error.
Desired Configuration Not Working as Expected
Try our Chrome extension to see what configuration the comment widget is being passed. If all fails, take as screenshot of what the chrome extension says and reach out to us.
Comments Missing on Same URL With Different Hash Bang
By default, FastComments will use the page URL for the "bucket" where comments are stored. If your URLs include #hashbangs
, and these #hashbangs
should not be part of the identifier that identifies a comment thread, we can simply ignore the hash bang value, for example:

Note that after making this change, a migration will have to be preformed for existing comments. For that, reach out to us.
URL Query Parameters Affecting Widget
By default, FastComments will use the page URL for the "bucket" where comments are stored. If your URLs include query parameters that should not be part of the identifier that identifies a comment thread, we can simply ignore them, for example:

Note that after making this change, a migration will have to be preformed for existing comments. For that, reach out to us.
Not Receiving Emails
At FastComments, we put a lot of work into ensuring our delivery of emails is as reliable as possible. However, some email providers are notoriously difficult to deliver to reliably. Check your spam folder for messages from fastcomments.com.
If you reach out to us we can usually provide more insight into why you may not be seeing emails from us.
Notion and Google Sites 
Want to add comments to your Notion pages? We have you covered.
Notion
For adding commenting to Notion docs, see the explanation video here.
Google Sites
Google Sites is also supported, and the flow is similar.
- Create a conversation to embed.
- When editing your Google Site, navigate to
Insert
->Embed
. SelectBy URL
. - Paste the URL from Step 1.
- Hit
Insert
. Done!
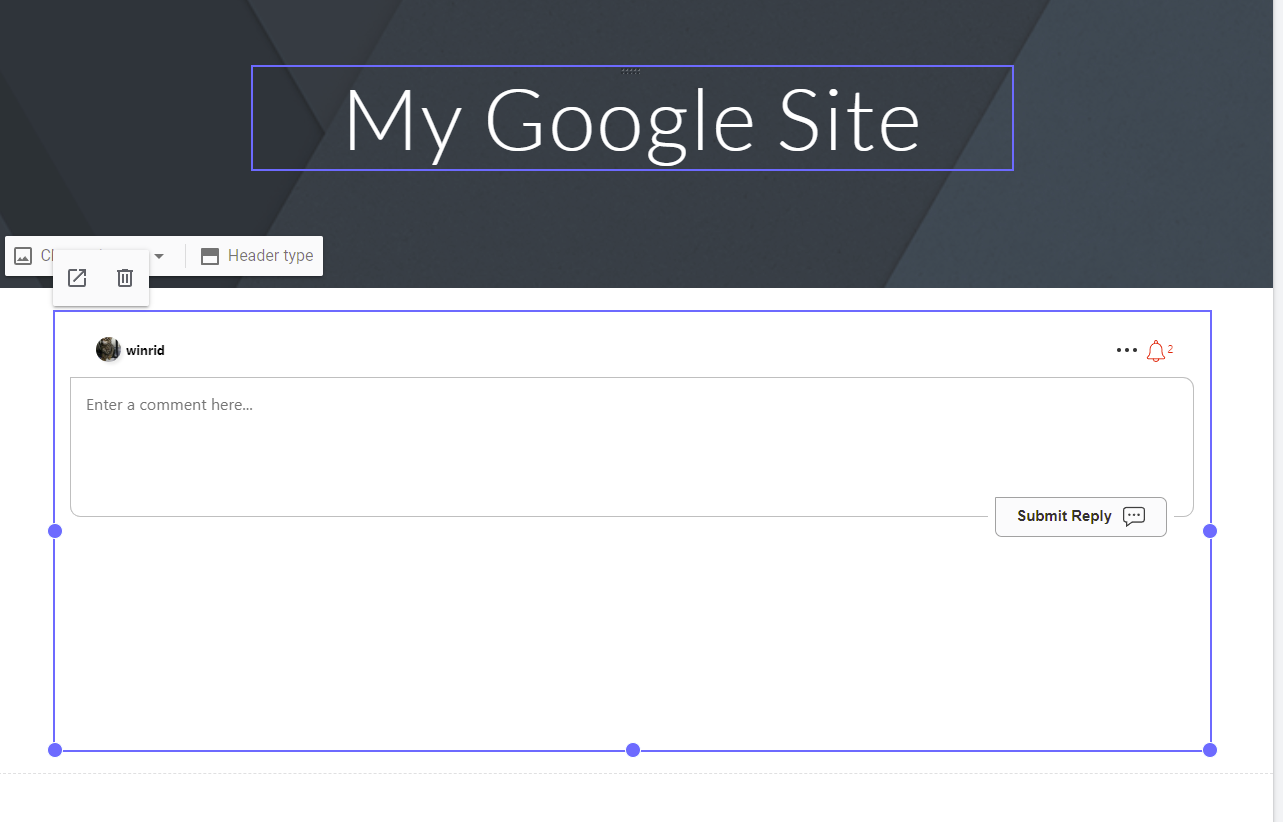
Nimbus Note
Adding live commenting to a Nimbus Note is also supported, and the flow is similar to Notion.
- Create a conversation to embed.
- When editing your note, add an embed block.
- Use the URL from Step 1 as the URL to embed.
- Done!